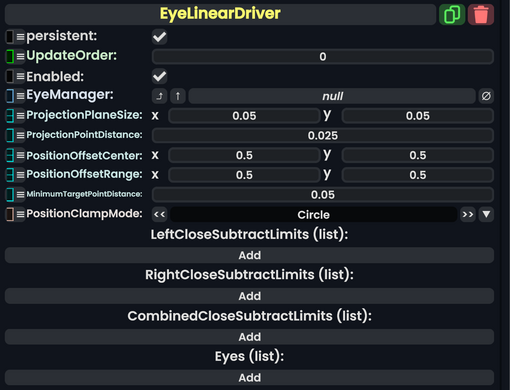
The EyeLinearDriver component is used to drive the shapekeys on eyes. It can also do eye look direction shapekeys if each eye is provided a projection plane slot.
This component can also be used to drive position offsets for eyes. This can be used to make 2D texture based eyes or anime eyes work without needing to use rotation. Please see How Projection Plane Works.
Fields
Name | Type | Description |
---|---|---|
persistent
|
Bool | Determines whether or not this item will be saved to the server. |
UpdateOrder
|
Int | Controls the order in which this component is updated. |
Enabled
|
Bool | Controls whether or not this component is enabled. Some components stop their functionality when this field is disabled, but some don't. |
EyeManager
|
EyeManager | The eye manager to get the eye data from. |
ProjectionPlaneSize
|
Float2 | The scaling of the inital hit point before it is clamped. See How Projection Plane Works. |
ProjectionPointDistance
|
Float | The distance from the offseted plane to ProjectionPlanePoint on all the eyes in Eyes list. See How Projection Plane Works.
|
PositionOffsetCenter
|
Float2 | How much to add to the inital plane projection hit point after clamping. See How Projection Plane Works. |
PositionOffsetRange
|
Float2 | how much to scale the inital plane projection hit after clamping. See How Projection Plane Works. |
MinimumTargetPointDistance
|
Float | The minimum distance for every eye in Eyes a look target point from that eye's projection plane slot can be. Points closer than that are set to be further than this value during calculation. See How Projection Plane Works.
|
PositionClampMode
|
EyeLinearDriver.ClampMode | How to clamp projection plane projection range when doing projection calculations. See How Projection Plane Works. |
LeftCloseSubtractLimits
|
list of EyeLinearDriver.EyeCloseLimit | The component finds the EyeCloseLimit with the maximum influence in this list. If the EyeCloseLimit with the maximum influence in CombinedEyeCloseSubtractLimits is higher, then that is used instead. Then this subtracts the result from the Left Eye close amount (essentially prying the eye open) to get the eye closed value to use with every left eye.
|
RightCloseSubtractLimits
|
list of EyeLinearDriver.EyeCloseLimit | The component finds the EyeCloseLimit with the maximum influence in this list. If the EyeCloseLimit with the maximum influence in CombinedEyeCloseSubtractLimits is higher, then that is used instead. Then this subtracts the result from the Left Eye close amount (essentially prying the eye open) to get the eye closed value to use with every right eye.
|
CombinedCloseSubtractLimits
|
list of EyeLinearDriver.EyeCloseLimit | Used with LeftCloseSubtractLimits and RightCloseSubtractLimits calculations.
|
Eyes
|
list of EyeLinearDriver.Eye | The list of eyes this component is doing calculations and shapekey driving for. |
Eye
Name | Type | Description |
---|---|---|
Side
|
EyeSide | The side to get data from for this Eye like target look point and |
ProjectionPlanePoint
|
Slot | The slot to use as a reference for doing Projection with. See How Projection Plane Works. |
PositionOffset
|
field drive of Float2 | The field to drive with the final value from the Projection system for fields like slot position or a 2D texture offset on a material. |
LookLeft
|
field drive of Float | Used to drive the surrounding eye surface shifting when the user looks left for this Eye. See Eye Look Calculation. |
LookUp
|
field drive of Float | Used to drive the surrounding eye surface shifting when the user looks up for this Eye. See Eye Look Calculation. |
LookRight
|
field drive of Float | Used to drive the surrounding eye surface shifting when the user looks right for this Eye. See Eye Look Calculation. |
LookDown
|
field drive of Float | Used to drive the surrounding eye surface shifting when the user looks down for this Eye. See Eye Look Calculation. |
OpenCloseTarget
|
field drive of Float | The shapekey or value to use when this eye is closed. |
PupilSizeTarget
|
field drive of Float | The shapekey or value to use when the pupil needs to grow. |
WidenTarget
|
field drive of Float | The shapekey to use to make this eye widen like in shock. |
SqueezeTarget
|
field drive of Float | The shapekey or value to use when this eye is squinting. |
FrownTarget
|
field drive of Float | The shapekey or value to use when this eye is making a frown. |
InnerBrowRaiseTarget
|
field drive of Float | The shapekey or value to use for the inner brow raising for this eye. |
InnerBrowLowerTarget
|
field drive of Float | The shapekey or value to use for the inner brow lowering for this eye. |
OuterBrowRaiseTarget
|
field drive of Float | The shapekey or value to use for the outer brow raising for this eye. |
OuterBrowLowerTarget
|
field drive of Float | The shapekey or value to use for the outer brow lowering for this eye. |
MinInputCloseness
|
Float | The minimum value the eye tracker can output for eye shut amount for this eye. The subtract limits are applied before the value from the eye tracker makes it to this filter. |
MaxInputCloseness
|
Float | The maximum value the eye tracker can output for eye shut amount for this eye. The subtract limits are applied before the value from the eye tracker makes it to this filter. |
OpenState
|
Float | The value to drive OpenCloseTarget to when the eye tracker is outputting the minimum possible value for eye shut amount. Which MinInputCloseness should also be set to the minimum possible value for eye shut amount from the eye tracker. The subtract limits are applied before the value from the eye tracker makes it to this filter.
|
CloseState
|
Float | The value to drive OpenCloseTarget to when the eye tracker is outputting the maximum possible value for eye shut amount. Which MaxInputCloseness should also be set to the maximum possible value for eye shut amount from the eye tracker. The subtract limits are applied before the value from the eye tracker makes it to this filter.
|
MinInputPupilSize
|
Float | The minimum value the eye tracker can output for pupil size for this eye. |
MaxInputPupilSize
|
Float | The maximum value the eye tracker can output for pupil size for this eye. |
MinOutputPupilSize
|
Float | The value to drive PupilSizeTarget to when the eye tracker is outputting the minimum possible value for pupil size. Which MinInputPupilSize should also be set to the minimum possible value for pupil size from the eye tracker.
|
MaxOutputPupilSize
|
Float | The value to drive PupilSizeTarget to when the eye tracker is outputting the maximum possible value for pupil size. Which MaxInputPupilSize should also be set to the maximum possible value for pupil size from the eye tracker.
|
MinInputWiden
|
Float | The minimum value the eye tracker can output for widen in shock for this eye. |
MaxInputWiden
|
Float | The maximum value the eye tracker can output for widen in shock for this eye. |
MinOutputWiden
|
Float | The value to drive WidenTarget to when the eye tracker is outputting the minimum possible value for widen in shock. Which MinInputWiden should also be set to the minimum possible value for widen in shock from the eye tracker.
|
MaxOutputWiden
|
Float | The value to drive WidenTarget to when the eye tracker is outputting the minimum possible value for widen in shock. Which MinInputWiden should also be set to the minimum possible value for widen in shock from the eye tracker.
|
MinInputSqueeze
|
Float | The minimum value the eye tracker can output for squinting for this eye. |
MaxInputSqueeze
|
Float | The maximum value the eye tracker can output for squinting for this eye. |
MinOutputSqueeze
|
Float | The value to drive SqueezeTarget to when the eye tracker is outputting the minimum possible value for squint. Which MinInputSqueeze should also be set to the minimum possible value for squint from the eye tracker.
|
MaxOutputSqueeze
|
Float | The value to drive SqueezeTarget to when the eye tracker is outputting the maximum possible value for squint. Which MaxInputSqueeze should also be set to the maximum possible value for squint from the eye tracker.
|
MinInputFrown
|
Float | The minimum value the eye tracker can output for frowning for this eye. |
MaxInputFrown
|
Float | The maximum value the eye tracker can output for frowining for this eye. |
MinOutputFrown
|
Float | The value to drive FrownTarget to when the eye tracker is outputting the minimum possible value for frown. Which MinInputFrown should also be set to the minimum possible value for frown from the eye tracker.
|
MaxOutputFrown
|
Float | The value to drive FrownTarget to when the eye tracker is outputting the maximum possible value for frown. Which MaxInputFrown should also be set to the maximum possible value for frown from the eye tracker.
|
MinInputInnerBrowRaise
|
Float | The minimum value the eye tracker can output for inner brow raising for this eye. |
MaxInputInnerBrowRaise
|
Float | The maximum value the eye tracker can output for inner brow raising for this eye. |
MinOutputInnerBrowRaise
|
Float | The value to drive InnerBrowRaiseTarget to when the eye tracker is outputting the minimum possible value for inner brow raising. Which MinInputInnerBrowRaise should also be set to the minimum possible value for inner brow raising from the eye tracker.
|
MaxOutputInnerBrowRaise
|
Float | The value to drive InnerBrowRaiseTarget to when the eye tracker is outputting the maximum possible value for inner brow raising. Which MaxInputInnerBrowRaise should also be set to the maximum possible value for inner brow raising from the eye tracker.
|
MinInputInnerBrowLower
|
Float | The minimum value the eye tracker can output for inner brow lowering for this eye. |
MaxInputInnerBrowLower
|
Float | The maximum value the eye tracker can output for inner brow lowering for this eye. |
MinOutputInnerBrowLower
|
Float | The value to drive InnerBrowLowerTarget to when the eye tracker is outputting the minimum possible value for inner brow lowering. Which MinInputInnerBrowLower should also be set to the minimum possible value for inner brow lowering from the eye tracker.
|
MaxOutputInnerBrowLower
|
Float | The value to drive InnerBrowLowerTarget to when the eye tracker is outputting the maximum possible value for inner brow lowering. Which MaxInputInnerBrowLower should also be set to the maximum possible value for inner brow lowering from the eye tracker.
|
MinInputOuterBrowRaise
|
Float | The minimum value the eye tracker can output for outer brow raising for this eye. |
MaxInputOuterBrowRaise
|
Float | The maximum value the eye tracker can output for outer brow raising for this eye. |
MinOutputOuterBrowRaise
|
Float | The value to drive InnerBrowOuterTarget to when the eye tracker is outputting the minimum possible value for outer brow raising. Which MinInputOuterBrowRaise should also be set to the minimum possible value for outer brow raising from the eye tracker.
|
MaxOutputOuterBrowRaise
|
Float | The value to drive InnerBrowOuterTarget to when the eye tracker is outputting the maximum possible value for outer brow raising. Which MaxInputOuterBrowRaise should also be set to the maximum possible value for outer brow raising from the eye tracker.
|
MinInputOuterBrowLower
|
Float | The minimum value the eye tracker can output for outer brow lowering for this eye. |
MaxInputOuterBrowLower
|
Float | The maximum value the eye tracker can output for outer brow lowering for this eye. |
MinOutputOuterBrowLower
|
Float | The value to drive InnerBrowOuterTarget to when the eye tracker is outputting the minimum possible value for outer brow lowering. Which MinInputOuterBrowLower should also be set to the minimum possible value for outer brow lowering from the eye tracker.
|
MaxOutputOuterBrowLower
|
Float | The value to drive InnerBrowOuterTarget to when the eye tracker is outputting the maximum possible value for outer brow lowering. Which MaxInputOuterBrowLower should also be set to the maximum possible value for outer brow lowering from the eye tracker.
|
LookMultiply
|
Float | See Eye Look Calculation. |
LookPower
|
Float | See Eye Look Calculation. |
EyeCloseLimit
ClampMode
Name | Value | Description |
---|---|---|
Circle
|
0 | clamps movement within an oval based on the Projection plane size. |
Square
|
1 | clamps movement within a rectangle based on Projection plane size. |
How Projection Plane Works
When any eye on this component is provided a slot for ProjectionPlanePoint
, the projection calculations are allowed to work.
For each eye the Calculation is able to be done on, the component shoots a simulated ray from the ProjectionPlanePoint
to the eye's look target through an offseted plane. The resulting hit point is modified by some of the component's values (See above fields) and then clamped between -1 to 1. Or within a circle if using ClampMode circle. The value is then modifed by some more component fields, before the result drives PositionOffset
. Which can drive a texture offset or the position of an eyeball slot for 2D eyes.
Eye Look Calculation
Eye look calculations for an eye don't work if ProjectionPlanePoint
is null for that eye.
The clamped hit point from The Projection System is first multiplied with LookMultiply
and raised to LookPower
before it is used for calculating LookLeft
, LookRight
, LookUp
, and LookDown
.
Usage
Can be used to drive 2D eyeballs, the shapekeys for eyeballs, and look shapekeys using Ray plane intersection calculations within the component.
Examples
Used in the eye management system added to avatars using the Avatar Creator if the option for settup eye tracking is enabled.