Component image 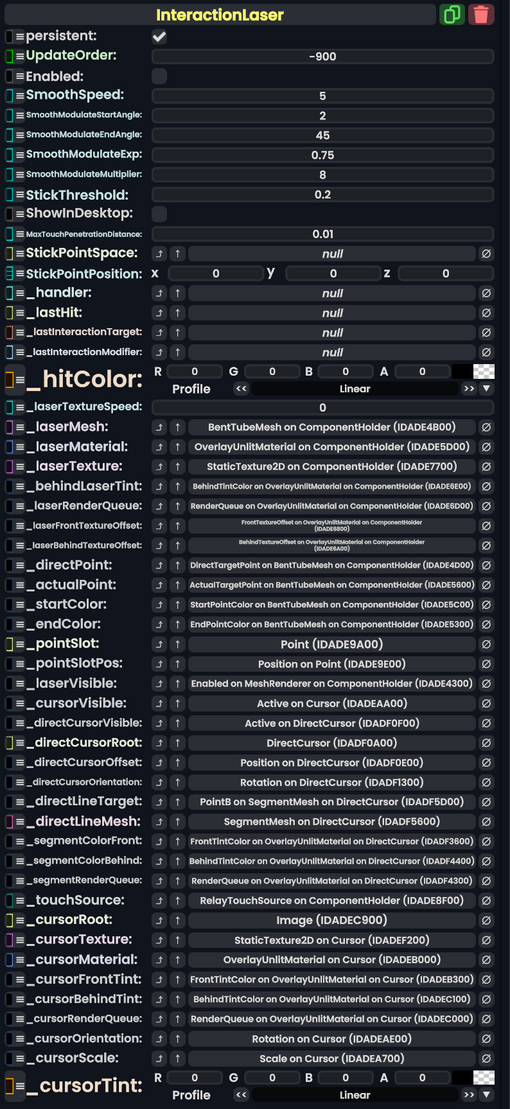
Interaction Laser component as seen in the Scene Inspector
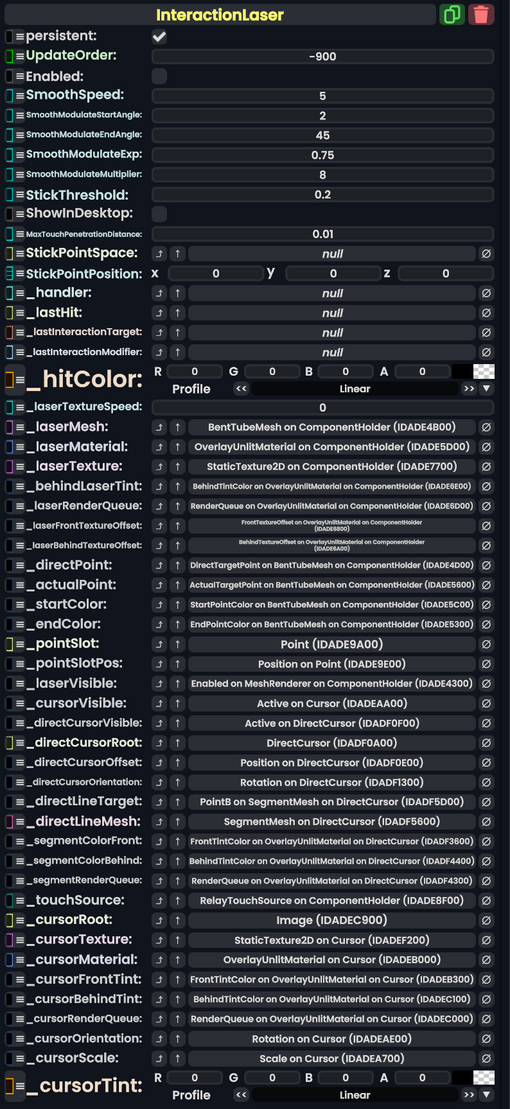
This article or section is a Stub. You can help the Resonite Wiki by expanding it.
Usage
Name | Type | Description |
---|---|---|
persistent
|
Bool | Determines whether or not this item will be saved to the server. |
UpdateOrder
|
Int | Controls the order in which this component is updated. |
Enabled
|
Bool | Controls whether or not this component is enabled. |
SmoothSpeed
|
Float | |
SmoothModulateStartAngle
|
Float | |
SmoothModulateEndAngle
|
Float | |
SmoothModulateExp
|
Float | |
SmoothModulateMultiplier
|
Float | |
StickThreshold
|
Float | |
MaxTouchPenetrationDistance
|
Float | |
StickPointSpace
|
Slot | |
StickPointPosition
|
Float3 | |
_handler
|
InteractionHandler | |
_lastHit
|
direct AutoSyncRef`1<Slot> | |
_lastInteractionTarget
|
direct AutoSyncRef`1<IInteractionTarget> | |
_lastInteractionModifier
|
direct AutoSyncRef`1<ILaserInteractionModifier> | |
_hitColor
|
ColorX | |
_laserTextureSpeed
|
Float | |
_laserMesh
|
BentTubeMesh | |
_laserMaterial
|
OverlayUnlitMaterial | |
_laserTexture
|
StaticTexture2D | |
_behindLaserTint
|
field drive of ColorX | |
_laserRenderQueue
|
field drive of Int | |
_laserFrontTextureOffset
|
field drive of Float2 | |
_laserBehindTextureOffset
|
field drive of Float2 | |
_directPoint
|
field drive of Float3 | |
_actualPoint
|
field drive of Float3 | |
_startColor
|
field drive of ColorX | |
_endColor
|
field drive of ColorX | |
_pointSlot
|
Slot | |
_pointSlotPos
|
field drive of Float3 | |
_laserVisible
|
field drive of Bool | |
_cursorVisible
|
field drive of Bool | |
_directCursorVisible
|
field drive of Bool | |
_directCursorRoot
|
Slot | |
_directCursorOffset
|
field drive of Float3 | |
_directCursorOrientation
|
field drive of FloatQ | |
_directLineTarget
|
field drive of Float3 | |
_directLineMesh
|
SegmentMesh | |
_segmentColorFront
|
field drive of ColorX | |
_segmentColorBehind
|
field drive of ColorX | |
_segmentRenderQueue
|
field drive of Int | |
_touchSource
|
RelayTouchSource | |
_cursorRoot
|
Slot | |
_cursorTexture
|
StaticTexture2D | |
_cursorMaterial
|
OverlayUnlitMaterial | |
_cursorFrontTint
|
field drive of ColorX | |
_cursorBehindTint
|
field drive of ColorX | |
_cursorRenderQueue
|
field drive of Int | |
_cursorOrientation
|
field drive of FloatQ | |
_cursorScale
|
field drive of Float3 | |
_cursorTint
|
ColorX |